In this article, we will learn how to send email using shell scripting. Sending emails programmatically can be very useful for automated notifications, error alerts, or any other form of communication that needs to be sent without manual intervention. Shell scripting offers a powerful way to achieve this through various utilities and tools available in Unix-like operating systems.
Prerequisites
- AWS Account with Ubuntu 24.04 LTS EC2 Instance.
- Basic knowledge of Shell scripting.
Step #1:Generate the App Password
First go to your account:
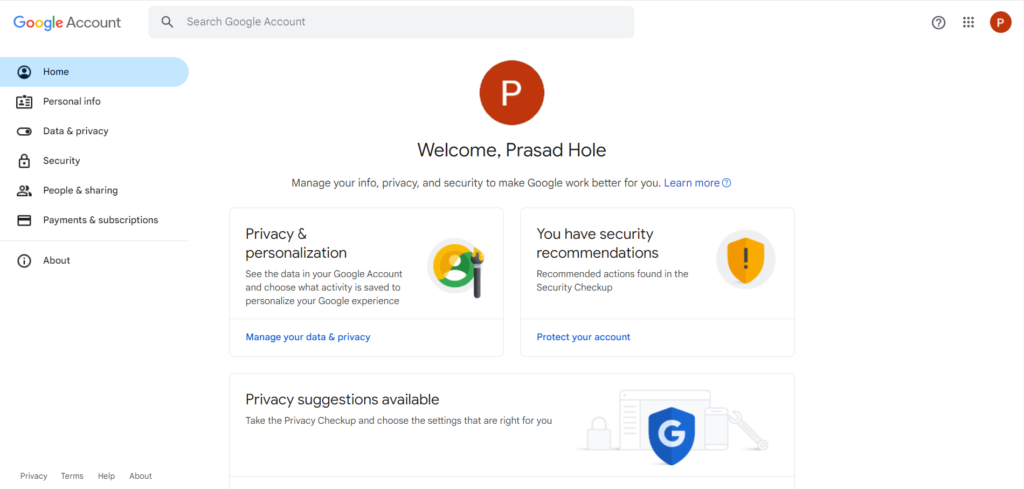
Search for App Password in the search bar.
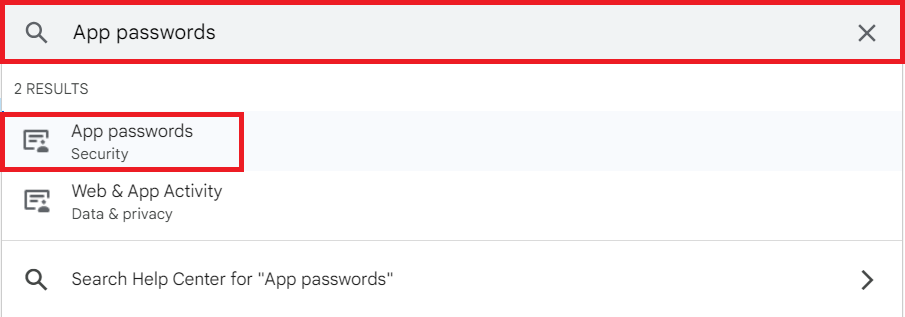
Enter your google account password to verify it’s you:
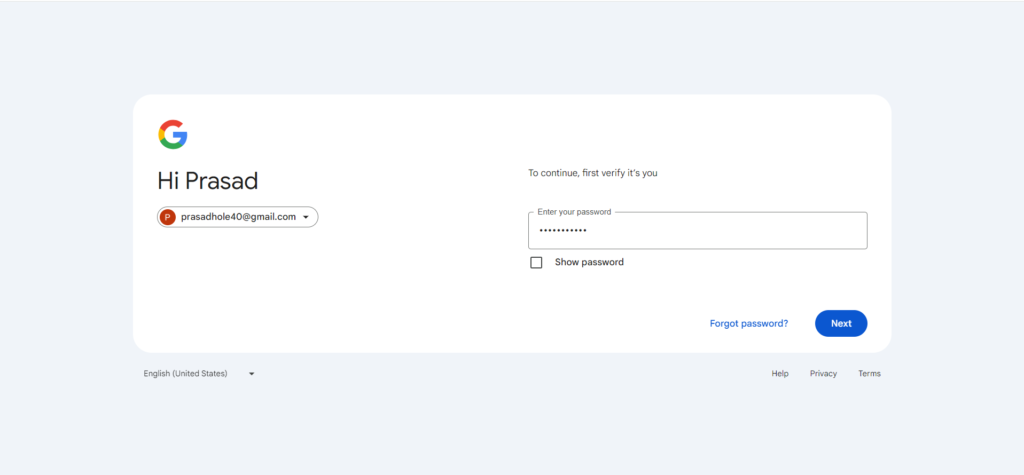
Now Enter the app name for which you wanted to get the app password like Shell Script.
And click on Create to create it:
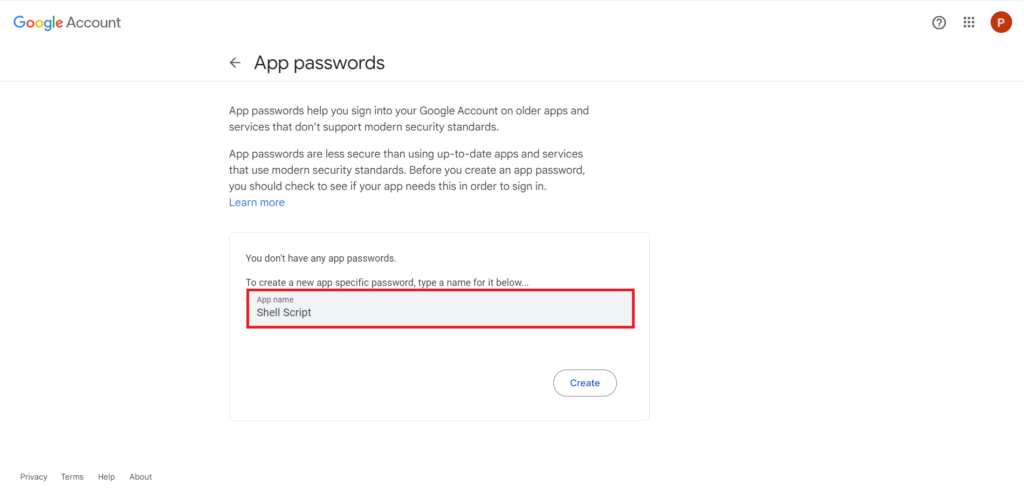
the password will be generated. Note it down cause will be using it.
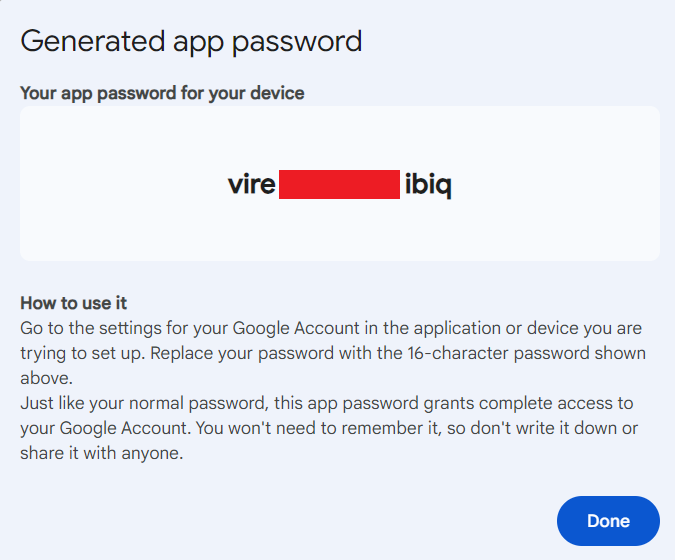
Step #2:Create a file in Ubuntu
Open the terminal and use the nano command to create a new file:
nano email.sh
Step #3:Write a Script to Send Emails
Below is a basic script to send an email.
-----------------------------------------
#!/bin/bash
# Prompt the user for input
read -p "Enter your email: " sender
read -p "Enter recipient email: " receiver
read -s -p "Enter your Google App password: " gapp
echo
read -p "Enter the subject of mail: " sub
# Read the body of the email
echo "Enter the body of mail (Ctrl+D to end):"
body=$(</dev/stdin)
# Sending email using curl
response=$(curl -s --url 'smtps://smtp.gmail.com:465' --ssl-reqd \
--mail-from "$sender" \
--mail-rcpt "$receiver" \
--user "$sender:$gapp" \
-T <(echo -e "From: $sender\nTo: $receiver\nSubject: $sub\n\n$body"))
if [ $? -eq 0 ]; then
echo "Email sent successfully."
else
echo "Failed to send email."
echo "Response: $response"
fi
------------------------------------------
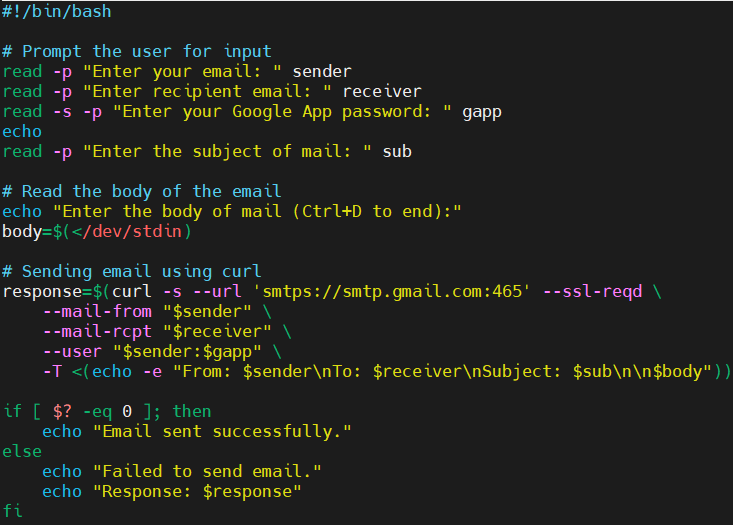
Save the file and exit the editor.
Explanation of the Script:
This script is designed to send an email using Gmail’s SMTP server through the command line. Here’s a step-by-step explanation of each part of the script:
- Shebang: The
#!/bin/bash
line at the beginning of the script indicates that the script should be run using the Bash shell. - User Input Prompts: These lines prompt the user to enter
- The sender’s email address.
- The recipient’s email address.
- The sender’s Google App password (entered silently using
-s
to hide the input). - The subject of the email.
- Reading the Email Body: This section prompts the user to enter the body of the email. The user can type the email content and end input by pressing
Ctrl+D
. The body of the email is then captured into the variablebody
. - Sending the Email: This part of the script uses
curl
to send the email-s
makescurl
run silently.--url 'smtps://smtp.gmail.com:465'
specifies the Gmail SMTP server with SSL on port 465.--ssl-reqd
ensures SSL is required.--mail-from "$sender"
specifies the sender’s email address.--mail-rcpt "$receiver"
specifies the recipient’s email address.--user "$sender:$gapp"
provides the sender’s email and Google App password for authentication.-T <(echo -e "From: $sender\nTo: $receiver\nSubject: $sub\n\n$body")
sends the email content including headers and body.
- Handling the Response: This final part checks the exit status of the
curl
command ($?
):- If the exit status is
0
, it prints “Email sent successfully.” - If the exit status is not
0
, it prints “Failed to send email.” and outputs the response fromcurl
.
- If the exit status is
Step #4:Make file executable
Change the file permissions to make it executable using the chmod command:
chmod +x email.sh
Step #5:Run the script
Run the script by executing the following command:
./email.sh
You will be prompted to enter the sender’s email, recipient’s email, Google App password, subject, and body of the email:
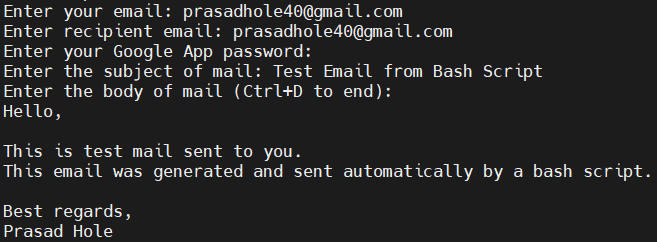
You will get the message Email sent successfully.
Now check the mail box to see if you receive the mail or not.
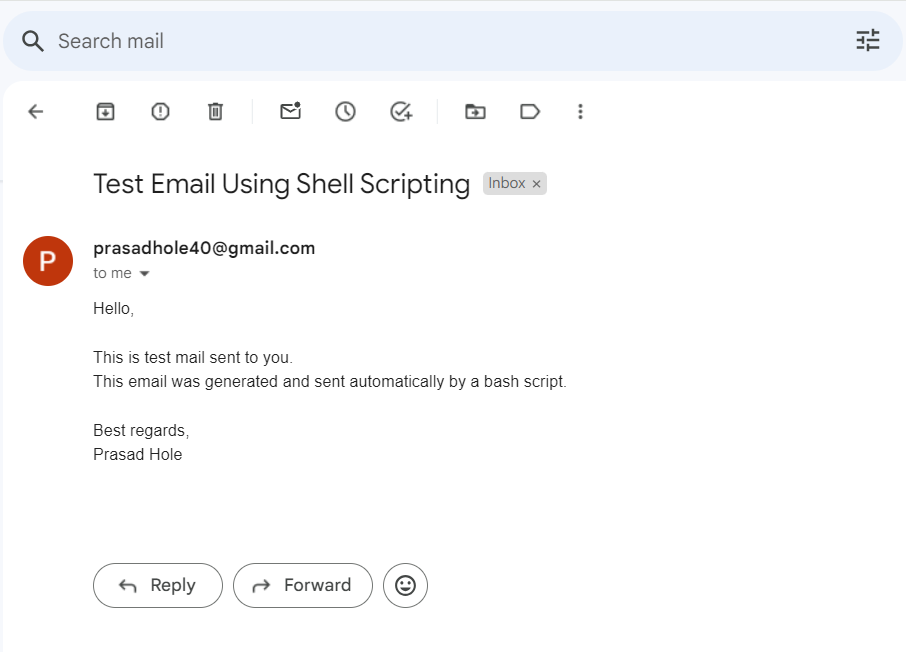
Conclusion:
In conclusion, sending emails using shell scripting can greatly enhance your automation tasks, enabling seamless communication and notifications. This approach is especially useful for automated notifications, error alerts, and other routine communications. With the power of shell scripting, you can integrate emails functionality into your workflows, ensuring timely and efficient information exchange.